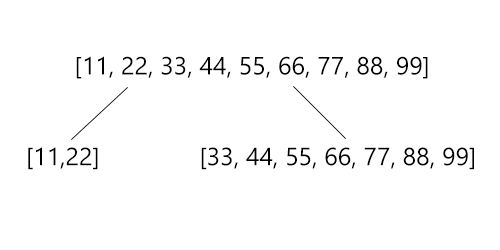
You can easily break an array containing N elements (where N>=2) into two (or more) separate arrays using the splice function.
Here’s an example on how you can do that:
const myArray = [11, 22, 33, 44, 55, 66, 77, 88, 99]
const segment1 = myArray.splice(0, 2)
const segment2 = myArray
console.log(segment1)
console.log(segment2)
Code language: JavaScript (javascript)
The above code would produce the following output:
[ 11, 22 ]
[
33, 44, 55, 66,
77, 88, 99
]
Code language: JSON / JSON with Comments (json)
Knowing from what index you want to break the arrays into parts, you can simply use the splice method. It’s worth noting that when you splice an array, as we did in this case, it returns the removed elements. We can then store these elements into another variable (segment1). The original array however (myArray) doesn’t contain the removed elements anymore. You can now either store this array into a new array (segment2) or use it as it is.
This Post Has One Comment