Earlier we saw how we can import one component into another in Svelte. But this is not of much use if we cannot pass some values to the components we import. After all, that’s an important part of any component. We can pass values to components in Svelte, almost similar to how we do it in React and Vue. However, there’s a difference in how we expose the settable variables to the outside world from our components.
Let’s have a look at this example to understand it better.
Here we have a component named CardComponent.svelte which contains some UI elements to show a card view:
<script>
export let name;
export let email;
</script>
<div>
<div class="crd">
<div class="crd-header">
{name}
</div>
<div class="crd-content">
{email}
</div>
</div>
</div>
<style>
.crd {
max-width: 300px;
max-width: 300px;
border: 1px solid #242424;
border-radius: 4px;
padding: 10px;
margin-top: 12px;
background: #fff;
}
.crd-header {
font-size: 18px;
font-weight: bold;
border-bottom: 1px solid #d5d5d5;
padding-bottom: 4px;
}
.crd-content {
font-size: 18px;
padding-top: 4px;
}
</style>
Code language: HTML, XML (xml)
You’d notice that on lines 2 and 3, we’re exporting two variables named name and email. Svelte uses these exported members as exportable props for this component. In other frameworks, we’d often need to define the props in the component with some special convention. However, with Svelte, it’s a bit different. Svelte leverages JavaScript’s inbuilt export keyword and uses the variable names to identify props.
Now, here’s our App.svelte component passing in the required props to the above component:
<script>
import CardComponent from './CardComponent.svelte'
let userName = 'Gautam'
let userEmail = 'test@example.com'
</script>
<div>
<h2>App component</h2>
<input bind:value="{userName}" />
<input bind:value="{userEmail}" />
<CardComponent name="{userName}" email="{userEmail}"/>
</div>
Code language: HTML, XML (xml)
You’d notice that not only are we passing states, but we’re passing states which are reactive in the current component.
Self-extending properties
One thing worth mentioning is that, in Svelte, we can also use self-extending properties as we do in React. It means that, if the prop name is the same as the property name in our parent component, then we can use a short-hand.
In the above example, if we used name and email in the parent component instead of userName and userEmail, then we can use the short-hand as follows:
<script>
import CardComponent from './CardComponent.svelte'
let name = 'Gautam'
let email = 'test@example.com'
</script>
<div>
<h2>App component</h2>
<input bind:value="{name}" />
<input bind:value="{email}" />
<CardComponent {name} {email}/>
</div>
Code language: HTML, XML (xml)
Therefore, instead of writing something like:
<CardComponent name="{name}" email="{email}"/>
Code language: HTML, XML (xml)
We could short it to:
<CardComponent {name} {email}/>
Code language: HTML, XML (xml)
Here’s what the above example looks like:
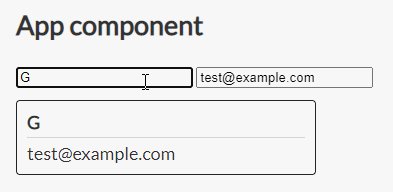