We can quickly load some HTML file content and show them unescaped in Vue 3. Let’s have a look at an example where we load some HTML content as string.
Let’s say we have a file named html-file.js containing some HTML string to be exported:
const htmlContent = `
<div>
<h1>Some sample title</h1>
<p>Some sample paragraph.</p>
</div>
`;
export default htmlContent
Code language: HTML, XML (xml)
And here’s our App.vue file importing and using the file content above:
<script setup>
import { ref, onMounted } from "vue";
import htmlFileContent from './html-file'
const htmlFileText = ref('')
onMounted(() => {
htmlFileText.value = htmlFileContent
})
</script>
<template>
<div>
<div v-html="htmlFileText">
</div>
</div>
</template>
Code language: HTML, XML (xml)
As we can see, we’ve imported the file and used it with our state htmlFileText. We’ve then bound the content with the v-html directive.
We can even bind multiple file contents to the same state and switch them by updating the state.
Here’s our App.vue component with two buttons, using one state to switch between two HTML content:
<script setup>
import { ref, onMounted } from "vue";
import htmlFileContent from './html-file'
import htmlFileContent2 from './html-file-2'
const htmlFileText = ref('')
onMounted(() => {
showFileContent1()
})
const showFileContent1 = () => {
htmlFileText.value = htmlFileContent
}
const showFileContent2 = () => {
htmlFileText.value = htmlFileContent2
}
</script>
<template>
<div>
<button @click="showFileContent1">Show file 1</button>
<button @click="showFileContent2">Show file 2</button>
<div v-html="htmlFileText">
</div>
</div>
</template>
Code language: HTML, XML (xml)
Here’s what the above file would look like in action:
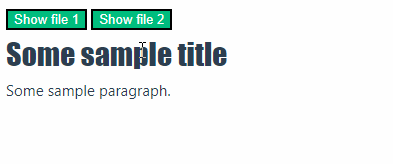