Vue is highly flexible as compared to other frameworks of same type. One of it’s biggest feature is it’s ability to access the root instance and derive methods or computed properties from it. Accessing the root instance is fairly simple in Vue. Here’s some code to demonstrate the same. Here’s the main.js file content:
import Vue from "vue";
import App from "./App.vue";
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App),
data() {
return {
appName: "Night"
};
}
}).$mount("#app");
Code language: JavaScript (javascript)
In the above code, I’ve added a data function with a default property of appName. I’ve assigned it’s value to “Night”. And here’s my App.vue component to access the root instance and it’s properties:
<template>
<div id="app">
<h2>Hello {{ rootName }}!</h2>
<small>wwww.nightprogrammer.com</small>
</div>
</template>
<script>
export default {
name: "App",
computed: {
rootName() {
return this.$root.appName;
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
}
</style>
Code language: HTML, XML (xml)
The output of the above code would be Hello Night!
You can also use it to access constants or methods. Here’s an example on how you can use it for constants:
This is the constants.js file containing some constant values:
export const appTitle = "Night programmer!";
export const appDescription = "Programming!";
export const appVersion = "1.0";
export const appAuthor = "Gautam";
const constants = {
appTitle: "Night programmer!",
appDescription: "Programming!",
appVersion: "1.0",
appAuthor: "Gautam"
};
export default constants;
Code language: JavaScript (javascript)
Here’s the main.js file which imports these constants and keeps after the created lifecycle hook is called:
import Vue from "vue";
import App from "./App.vue";
import constants from "./constants";
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App),
data() {
return {
appName: "Night"
};
},
created() {
this.constants = constants;
}
}).$mount("#app");
Code language: JavaScript (javascript)
And finally, here’s the App.vue file which can directly access the constants now. Note that you can access these constants in any file you want.
<template>
<div id="app">
<h2>Hello {{ rootName }}!</h2>
<p>App description: {{ constants["appDescription"] }}</p>
<p>App author: {{ constants["appAuthor"] }}</p>
<p>App verion: {{ constants["appVersion"] }}</p>
<small>wwww.nightprogrammer.com</small>
</div>
</template>
<script>
export default {
name: "App",
computed: {
rootName() {
return this.$root.appName;
},
constants() {
return this.$root.constants;
},
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
}
</style>
Code language: HTML, XML (xml)
The output of the above file would look something like this:
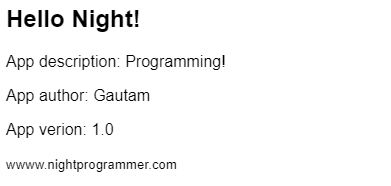
You can find a working demo of the above code from my repos below: