In JavaScript, there are multiple popular data manipulation libraries like lodash, underscore, buryjs, etc. However, there’s a huge community who oppose the usage of such libraries, including me. I mean, Babel does a fantastic job compiling all your ES6 / ES2015+ code into native JS. And since ES6 has almost all the functionality you’d use in lodash, I think using these libraries is an overkill. There is, in fact, an NPM library called you-dont-need-lodash-underscore. A little configuration in your ES Lint can make almost all the lodash methods accessible in your native JS code.
Here I’m going to show you how to sort a list of data from a table using native JS only.
Project code
Here’s the template code:
<template>
<div>
<h2>Sortable list 📋</h2>
<table>
<tr>
<th @click="sortList('id')">ID ↕</th>
<th @click="sortList('name')">Name ↕</th>
<th @click="sortList('email')">Email ↕</th>
</tr>
<tr v-for="(data, index) in sortedData" :key="index">
<td>{{ data.id }}</td>
<td>{{ data.name }}</td>
<td>{{ data.email }}</td>
</tr>
</table>
<div style="padding-top: 10px">wwww.nightprogrammer.com</div>
</div>
</template>
Code language: HTML, XML (xml)
You’d notice that at every th (Table Head), I’ve binded a menthod named sortList() with the @click event listenener. I’ve also passed the column name to the method. Now, here’s the script code to handle the functionality:
<script>
export default {
name: "ItemList",
computed: {
originalData() {
return [
{ id: 1, name: "Night", email: "nightprogrammer95@gmail.com" },
{ id: 2, name: "Gautam", email: "mailgautam@test.com" },
{ id: 3, name: "Alex", email: "xalex@testmail.com" },
{ id: 4, name: "Zora", email: "zora@mail.com" },
{ id: 5, name: "Peter", email: "peter.me@test.com" },
];
},
},
data() {
return {
sortedData: [],
sortedbyASC: true,
};
},
mounted() {
this.sortedData = this.originalData;
console.log(this.sortedData);
},
methods: {
sortList(sortBy) {
if (this.sortedbyASC) {
this.sortedData.sort((x, y) => (x[sortBy] > y[sortBy] ? -1 : 1));
this.sortedbyASC = false;
} else {
this.sortedData.sort((x, y) => (x[sortBy] < y[sortBy] ? -1 : 1));
this.sortedbyASC = true;
}
},
},
};
</script>
<style>
table {
font-family: arial, sans-serif;
border-collapse: collapse;
width: 100%;
}
td,
th {
border: 1px solid #dddddd;
text-align: left;
padding: 8px;
}
th:hover {
cursor: pointer;
background: rgb(229, 255, 211);
}
tr:nth-child(even) {
background-color: #f3f3f3;
}
</style>
Code language: HTML, XML (xml)
As you can see, I’ve got a computed property called originalData where I’m keeping a list of data. Then, when the sortList() method is called (on line 27), I’m first checking if the list is already sorted in ascending order. sortedByASC is true by default. Now, if it’s true, I’m reversing the list using the sort() method on line 28. For each pair of array elements, 1st and 2nd in first case, if the 1st is greater than the 2nd, then I return -1, otherwise 1. A positve value swaps the pairs (1st with 2nd), and a negative value skips it. Once the swapping is done throughout the list, I then set the sortedByASC value to false. This is because the list is now sorted in descending order. The reverse happens on line 31.
The view for the above code should look like this:
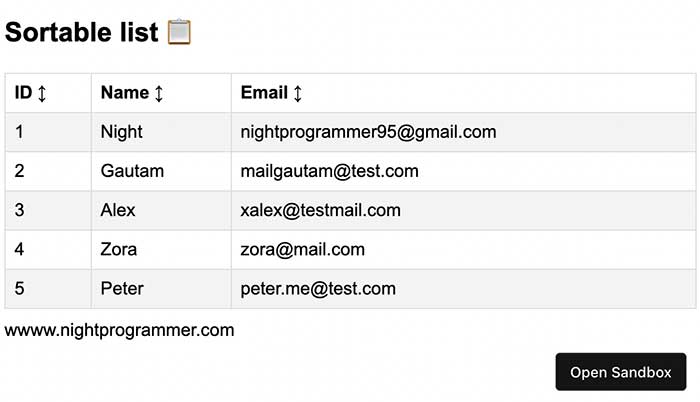
You can find a working demo from my repos here: