We can v-model a value in the child component with the modelValue directly. Consider a button in the parent component bound with a boolean reactive state. This reactive state can be directly bound to the child component with the v-model being passed to the child component. The child component can then access its value using the modelValue prop.
Here’s the ParentComponent.vue file with a reactive state called childComponentState.
<script setup>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
const childComponentState = ref(false)
const toggleModelValue = () => {
childComponentState.value = !childComponentState.value
}
</script>
<template>
<div>
<h2>Parent component</h2>
<button @click="toggleModelValue">Change model value</button>
<ChildComponent v-model="childComponentState"/>
</div>
</template>
Code language: HTML, XML (xml)
And here’s the ChildComponent.vue file which receives the modelValue and toggles some text based on it:
<script setup>
import { reactive } from 'vue';
const props = defineProps({
modelValue: {
type: Boolean,
default: false
}
})
</script>
<template>
<div>
<div class="child">
<h3>
Child component
</h3>
<div v-if="modelValue">
<strong>
Model value is true! ✅
</strong>
</div>
<div v-else>
<strong>
Model value is false! ❌
</strong>
</div>
</div>
</div>
</template>
<style scoped>
.child {
border: 1px solid #00bd7e;
margin: 10px 0;
padding: 24px;
}
</style>
Code language: HTML, XML (xml)
As you can see in the above code, we’re using defineProps and binding the v-model directly with the modelValue prop. This modelValue prop is reactive to the state (childComponentState) we passed from the parent component.
Here’s how the above code would look like in action:
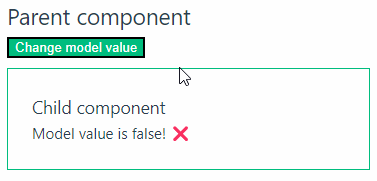