Earlier we’ve seen how we can use modelValue to react to a parent state in a child component. Now, let’s have a look at how we can change the value of a state in the parent component using the update:modelValue event emitter from the child component.
Here’s our ParentComponent.vue file containing the child component:
<script setup>
import { ref } from 'vue';
import ChildComponent from './ChildComponent.vue';
const childComponentState = ref(false)
const setModelValueTrue = () => {
childComponentState.value = true
}
</script>
<template>
<div>
<h2>Parent component</h2>
<button @click="setModelValueTrue">Set model value true</button>
<ChildComponent v-model="childComponentState"/>
</div>
</template>
Code language: HTML, XML (xml)
And here’s our ChildComponent.vue file from within which, we can now change the value of the reactive state childComponentState.
<script setup>
const props = defineProps({
modelValue: {
type: Boolean,
default: false
}
})
const emit = defineEmits(['update:modelValue'])
</script>
<template>
<div>
<div class="child">
<h3>
Child component
</h3>
<div v-if="modelValue">
<strong>
Model value is true! ✅
</strong>
</div>
<div v-else>
<strong>
Model value is false! ❌
</strong>
</div>
<button @click="$emit('update:modelValue', false)">
Set model value false
</button>
</div>
</div>
</template>
<style scoped>
.child {
border: 1px solid #00bd7e;
margin: 10px 0;
padding: 24px;
}
</style>
Code language: HTML, XML (xml)
As we can see in the above file, we’ve first defined the modelValue prop on line 3. We’ve then defined the emit for updating the model value at line 8. We’ve finally updated the model value with a button click and set its value to false on line 27.
Here’s what the above code would look like in action:
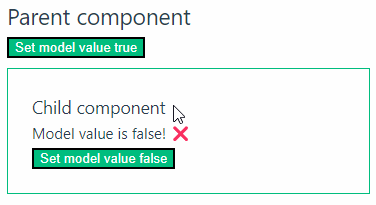
Hi, how to do it with a property which is NOT v-model/modelValue, but i.e. ‘myprop’? At my project your code works fine, but no longer reflects changes back to parent when I use a custom name for the property. Thanks!