It’s always a good idea to keep all your strings in one file and access them across your templates and js/ts files. You can of course use the data() method given by Vue.js to store some strings. But there’s a problem with that approach.
<template>
<div id="app">
<h1>{{ myTitle }}</h1>
<h3>{{ myDescription }}</h3>
<div class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
myTitle: "Some title",
myDescription: "Some description",
};
},
};
</script>
Code language: HTML, XML (xml)
You can use some strings like the code below. But the problem is, you’re storing some values in states which are reactive. This means, Vue would internally attach observers to these variables to detect changes. However, since these are string constants, they’ll never change. We’re, indirectly creating states which will always have a single state only.
A better way is to store all the string constants in a file and install it in Vue as a Vue plugin. Here’s how you can do it:
// strings.js
export const myTitle = "Hello!";
export const myDescription = "Hello from nightprogrammer.com!";
Code language: JavaScript (javascript)
// string-constants.js
import * as allStrings from "./strings";
const stringConstants = {
...allStrings
};
stringConstants.install = function (Vue) {
Vue.prototype.$stringConstants = (key) => {
return stringConstants[key];
};
};
export default stringConstants;
Code language: JavaScript (javascript)
// main.js
import Vue from "vue";
import App from "./App.vue";
import stringConstants from "./string-constants";
Vue.use(stringConstants);
Vue.config.productionTip = false;
new Vue({
render: (h) => h(App)
}).$mount("#app");
Code language: JavaScript (javascript)
With the above setup, you can now access the strings in any component easily:
// App.vue
<template>
<div id="app">
<h1>{{ $stringConstants("myTitle") }}</h1>
<h3>{{ $stringConstants("myDescription") }}</h3>
<div class="np-credits">wwww.nightprogrammer.com</div>
</div>
</template>
<script>
export default {
name: "App",
};
</script>
Code language: HTML, XML (xml)
You can also access the strings in the script by using the this.$stringConstants(‘stringName‘) accessor.
Here’s how the output should look like:
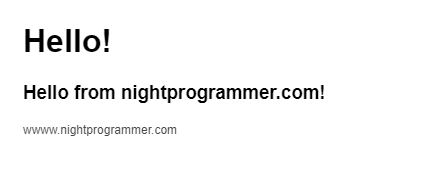
Your blog is a gem for Vue.js developers like us! I can’t thank you enough for all the effort you put in here. .. Just keep up the great work!!