Earlier we’ve seen how we can generate and place a random custom color avatar in Vue.js with name initials. In this tutorial, we’ll have a look at how we can quickly create an avatar from names using a library.
First of all, install the library with the following command:
npm i vue-avatar
Once installed, you can now import and use the component as needed:
<template>
<div id="app">
<div class="avatar-container">
<avatar inline username="Gautam Kabiraj"></avatar>Gautam Kabiraj
</div>
<div class="avatar-container">
<avatar inline username="Tom Smith"></avatar>Tom Smith
</div>
<div class="avatar-container">
<avatar inline username="Lucy Frank"></avatar>Lucy Frank
</div>
<div class="avatar-container">
<avatar inline username="Mona Lisa"></avatar>Mona Lisa
</div>
</div>
</template>
<script>
import Avatar from "vue-avatar";
export default {
name: "App",
components: {
Avatar,
},
};
</script>
<style>
#app {
font-family: "Avenir", Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
color: #000000;
margin-top: 60px;
}
.avatar-container {
padding: 8px;
}
</style>
Code language: HTML, XML (xml)
Now you’ll see the avatars with initials showing up in your Vue app.
Of course, you can pass in a few other props to customize the avatar as follows:
inline: It’ll make the component display type inline
src: It’ll show the image inside the avatar
customStyle: It’ll override the CSS styles with your own
backgroundColor: It’ll set the given background color
color: It’ll set the given color
lighten: It’ll lighten the background color(-100 to 100)
size: To set the avatar size in pixels
rounded: To keep the avatar rounded. You can set it to false as well.
parser: It’ll parse an input string
This is how it should look like in your screen:
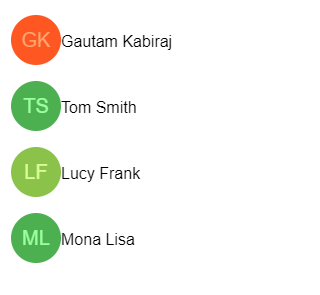
You can find a working demo from my repo links below: