Like any other SPA framework, Svelte allows us to show parts of HTML or components conditionally. If we compare it with React, in React we return a fragment/component for each of the conditions met. In Vue, we show a component or HTML based on the truthiness of the first line of the segment.
However, here in Svelte, we can conditionally show HTML inside the if-else block like a regular JavaScript expression with a slight syntactic enhancement.
Let’s have a look at an example to understand this better. In this example, we’d tell the user how strong the entered password is, based on the length of the input. If the input length is greater than 7, we show ‘strong’. If it is greater than 4 but less than 7, we show ‘moderate’. And if it is less than 4, we show ‘poor’.
Here’s the demo code:
<script>
let password = ''
$: passwordLength = password.length
</script>
<div>
<input
type="password"
bind:value={password}
placeholder="Enter password"
class="password"
/>
<p>
{#if passwordLength > 7}
<span>? Strong</span>
{:else if passwordLength > 4}
<span>? Moderate</span>
{:else}
<span>? Poor</span>
{/if}
<span> password! </span>
</p>
</div>
<style>
.password {
font-size: 22px;
outline: none;
}
</style>
Code language: HTML, XML (xml)
As we can see in the above example, we’ve got a bunch of if-elseif-else statements to display different kinds of HTML.
Here’s what the above example would look like in action:
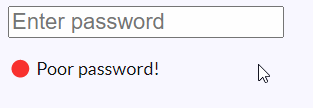