In this tutorial, we will learn how to create an iOS-style toggle switch button purely in Vue.js without using any library.
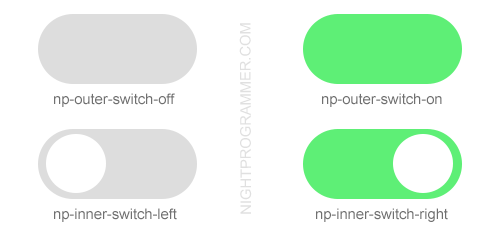
Here’s the underlying concept of the functionality we’re going to implement:
- Create an outer div with a default background color for the disabled state. We can change the background color of this div depending on the active property of the state. We do this by adding another class containing the active background color.
- Create an inner div with a position left aligned relative to the outer div. We change the position of the inner element by adding another CSS class with some left spacing.
Here’s the code to implement the functionality:
<template>
<div>
<h3>Toggle switch {{ isEnabled ? "ON" : "OFF" }}</h3>
<div
@click="toggleSwitch"
:class="{
'np-outer-switch': true,
'np-outer-switch-on': isEnabled,
'np-outer-switch-off': !isEnabled,
}"
>
<div
:class="{
'np-inner-switch': true,
'np-inner-switch-left': !isEnabled,
'np-inner-switch-right': isEnabled,
}"
></div>
</div>
</div>
</template>
<script>
export default {
name: "App",
data() {
return {
isEnabled: false,
};
},
methods: {
toggleSwitch() {
this.isEnabled = !this.isEnabled;
},
},
};
</script>
<style>
#app {
font-family: Avenir, Helvetica, Arial, sans-serif;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
text-align: center;
color: #0066ff;
margin: 60px;
max-width: 400px;
}
h3 {
text-align: left;
}
.np-outer-switch {
width: 70px;
height: 34px;
border-radius: 30px;
background: rgb(212, 212, 212);
cursor: pointer;
position: relative;
}
.np-outer-switch-off {
background: rgb(212, 212, 212);
transition: background 0.4s;
}
.np-outer-switch-on {
background: rgb(0, 202, 51);
transition: background 0.4s;
}
.np-inner-switch {
height: 30px;
width: 30px;
background: #fff;
border-radius: 50%;
position: absolute;
top: 2px;
left: 2px;
}
.np-inner-switch-left {
top: 2px;
left: 2px;
transition: left 0.4s;
}
.np-inner-switch-right {
top: 2px;
left: 38px;
transition: left 0.4s;
}
</style>
Code language: HTML, XML (xml)
In the above code, we’ve bound a toggleSwitch method to the div on line 5. This method toggle’s the boolean value of isEnabled reactive state on line 28,
Depending on the truthiness of this reactive state, we append/remove multiple CSS classes.
We toggle multiple CSS classes through lines 6-10 for the outer div. And multiple CSS classes through lines 13-17 for the inner div.
Here’s what the above code would look like in action:
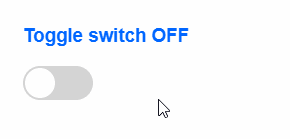
You can find a working version of the above code from my repos below: