Writing your first application in Vue.js is a breeze. There are multiple ways to write Hello World in Vue.js. Since it’s a JavaScript framework, you can easily test a simple application in a online platform. Alternatively, you can write in your own set-up environment using an IDE, of course. You’ll most likely be using some offline IDEs to develop your projects using Vue.js later. Let’s look at all the possible ways to write a Vue.js application one by one.
1. Using online code editor
Go to jsfiddle (https://jsfiddle.net/) or codepen (https://codepen.io/) to start writing your first application. I’m going to use codepen for this example.
Go to the following link in another tab (https://vuejs.org/v2/guide/) and copy the Vue.js script code which may look like this:
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
Code language: HTML, XML (xml)
You’ll see there are two versions of Vue.js available. One for development purpose and another for production use. The difference is very simple. In the development version, you’ll have the option to see warnings, messages, suggestions and detailed error logs whenever something goes wrong. In the production version, you’re pretty much on your own. Of course, you’ll still get errors but not as detailed to debug. Another difference is the code structure. The production version is a minified gziped JavaScript. So, it’s definitely smaller and faster to load and execute than the development counterpart. I’d recommend you to choose the development version while learning and debugging. You can of course switch to production when you’re about to release your production-ready application.
With the link copied, you can switch back to codepen and in the HTML window, paste the copied code inside the body tag like this:
<title>Hello World</title>
</head>
<body>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</body>
Code language: HTML, XML (xml)
With that in place, you’re ready to write your first bit of code.
Add the following <div> element to your body tag:
<div id="hello">
<h1>{{ message }}</h1>
</div>
Code language: HTML, XML (xml)
So that the complete HTML looks like this:
<head>
<title>Hello World</title>
</head>
<body>
<div id="hello">
<h1>{{ message }}</h1>
</div>
<script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script>
</body>
Code language: HTML, XML (xml)
In the above code, anything kept between {{ and }} is filled by Vue.js. This is called the interpolation text. When the DOM renders, Vue.js looks for the target element (in this case, it is the id hello). Once it finds the target element, it then tries to find some variables. These are not ordinary variables, they must be defined in the Vue instance. Once it finds those variables, it searches for the values that it can replace the variables (defined in the Vue instance) with. Right now, that variable is message. Now where does the value “Hello World!” in message comes from? Of course, the Vue instance. But what is a Vue instance? Let’s have a deeper look.
Add the following JavaScript code to the JS window:
var vue_ins = new Vue({
el: ‘#hello’,
data: {
message: ‘Hello World!’
}
});
Code language: PHP (php)
In the above code, you’re creating a Vue instance using new Vue(). Inside the Vue() method, you can supply multiple parameters. One mandatory parameter is the el parameter. el stands for element that you want Vue.js to target. It should be an element id that you’re using in your HTML code. For example, you’re using the hello id here. Additionally, you can also pass data to the target element through variables. You must define the variable names and their default values inside the data object first. Whatever variables you are going to use in the HTML. For example, here you’re using the message variable with a default value set to ‘Hello World!’. You must keep in mind that the Vue instance can take much more than just data variables. It can hold user-defined methods, lifecycle methods, computed values, and much more. You’ll explore all of these features shortly.
And that’s it! You should now see hello world in your browser!
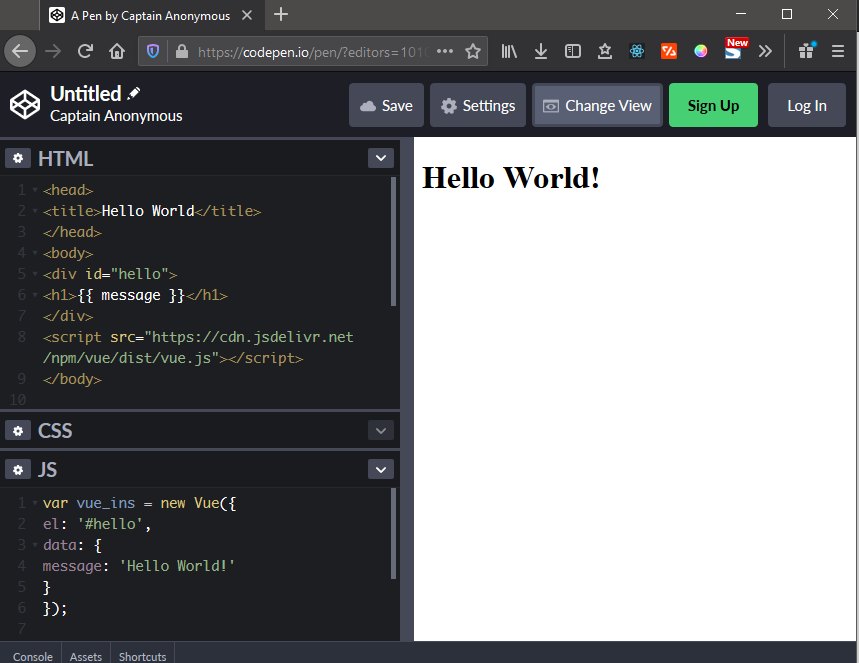
That was how you could write your very first Vue.js application in an online editor. Next, let’s try to write a Vue.js application using NPM (Node Package Manager). You’ll also have a deeper dive at some of the Vue.js terms we’ve used so far.
2. Using Vue CLI
This is the type that you’re going to use for large-scale applications, and in a real-world development environment.
Before installing Vue.js on your system, you must make sure that you have Node.js installed. If you don’t have Node.js, you can simply head over to its official website https://nodejs.org/en/ and download the latest version from there. You’ll also have NPM auto-installed with Node.js. If not, keep an eye while running the Node.js setup in case it asks for NPM installation separately.
After installing Node.js, you’re going to create a folder anywhere for your Vue.js application. Let’s create one on our desktop and name it Vue Hello. Now, go inside the folder, press and hold the Shift key, right click your mouse and select “Open command window here”. Mac or Linux users can open the Terminal here.
Now, type in the following command and hit the Enter key / Return key.
npm install vue
This will install the latest Vue.js version on your machine. Wait for the process to finish. By the end, you’ll have something like this:
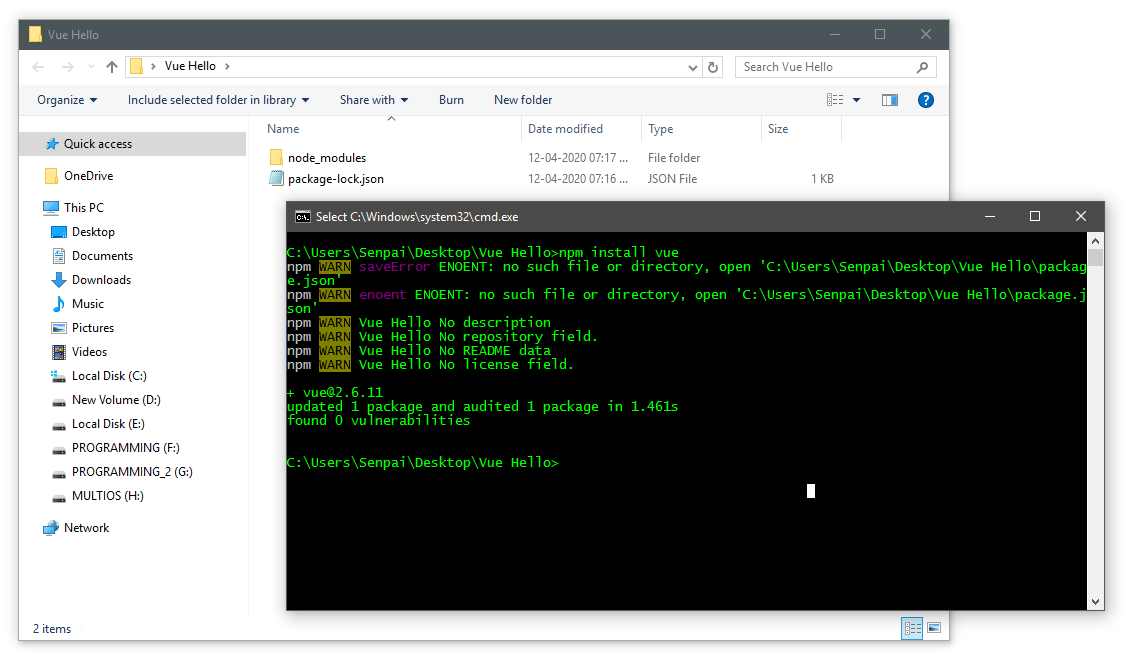
You’ll notice that that after the process finish, it’ll show the Vue.js version it has installed at the bottom (vue@2.6.11). By the time I’m writing this article, it is v2.6.11 but you’ll most likely have a newer version.
After installing Vue.js, you need to install the Vue CLI (Vue Command Line Interface). It is something that enables you to execute various Vue commands using the command line. Remember, the Vue we installed just now only contains the library. It doesn’t know how to bundle, execute, or run a Vue.js server to start an application. That’s why you need the Vue CLI.
So go ahead and run the following command:
npm install @vue/cli
Code language: CSS (css)
You can optionally add the –global flag to the command to make it accessible from anywhere on your machine (npm install @vue/cli –global). This will install the Vue CLI.
After installing Vue CLI, it’s time to create a Vue.js application. So, type in the following command to install Vue.js with the Webpack configuration enabled:
vue init webpack vue-hello
Here, we’re calling our app name “Vue Hello” by using vue-hello in the command. It’ll ask us a bunch of questions. We can either type in the details or hit Enter key to keep in the defaults. We may also be expected to choose among some options for some queries. It’ll ask us to answer things like:
Project name: Here we can type the name of the project
Project description: Here we can give a short description about the project
Author: Here we can write our own name or the company’s number under which we’re delivering the project
Vue build: We can keep it to default, that is standalone (which means one single JavaScript file for the final build).
Install vue-router: We will, almost in all the Vue projects, need a router. So it’s a good idea to select Yes here.
Use ESLint to lint your code: We often need a Linter to improve our code quality and make it error-free. So, Yes.
Pick an ESLint preset: If we chose Yes in the previous menu, we’ll be asked to select a preset. A preset here is a set of configurations already defined and used by some of the more popular companies. Here we choose Airbnb. You can select something else if you wish to.
Set up unit tests: Here we can decide if we want to have custom unit tests for the project. Right now, we’re only learning the basics and ain’t interested in testing so, No.
Setup e2e tests with Nightwatch: Here we can select if we need an End-To-End testing librabry like Nightwatch. Right now, it’s not our thing but I’d definitely suggest you to check it out sometime later. After advancing a bit in Vue.js, of course.
Should we run ‘npm install’ for you after the project has been created (recommended): Select npm. This will help us auto-install all the required packages used by our application.
After filling all these, hit the Enter key and wait for the process to finish.
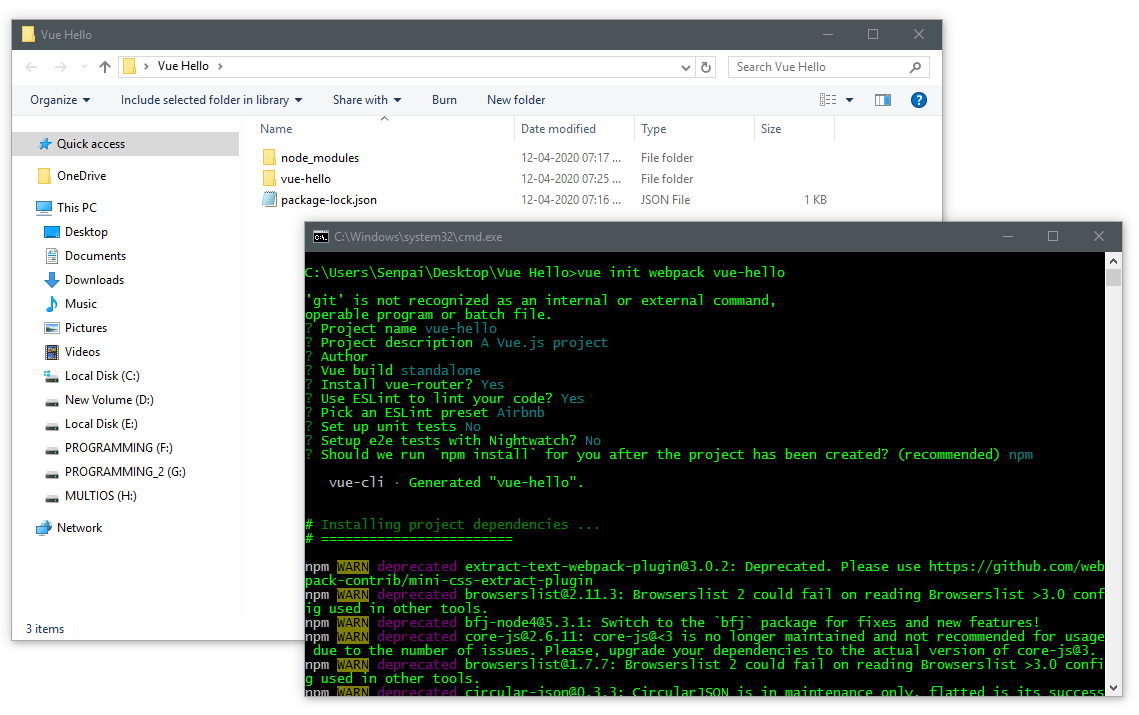
At the end of the process, you’ll notice a new folder created by the name of your application (vue-hello).
Now, type in the following commands one by one:
cd vue-hello
npm install
npm run dev
You should now have your application running at a given port address in your local server. In my case, it is at 8080. Open your web browser and go the the said address and you should see something like this:
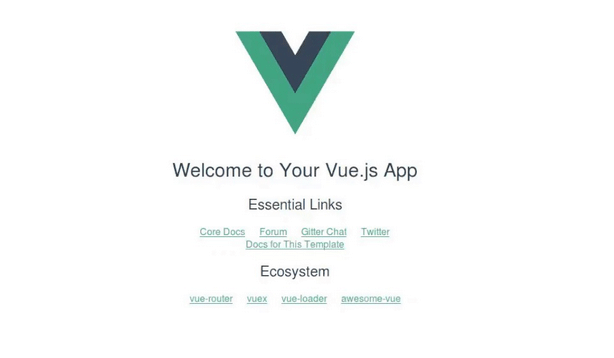
Now, open the project folder (Vue Hello) in your favorite code editor. You can use whatever you use like VS Code, Sublime, Atom, XCode, etc. I’m using VS Code. You’ll notice the following project structure already created for you by Vue CLI:
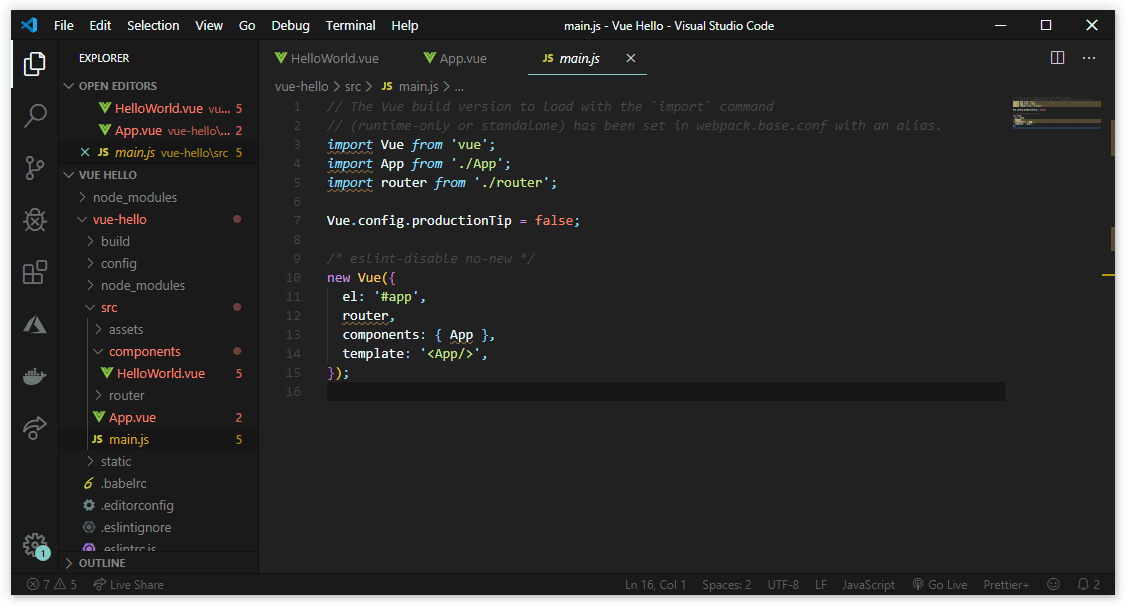
Navigate to the /src folder and open main.js file. This is the entry point of the application you’re looking at. Here you can define all sort of ingredients that goes into the application wherever required. Here, you’ll notice something known, right, the Vue instance.
import Vue from ‘vue’;
import App from ‘./App’;
import router from ‘./router’;
new Vue({
el: ‘#app’,
router,
components: { App },
template: ‘<App/>’,
});
Code language: PHP (php)
In the above code, you’re importing the Vue library, the App component from the App.vue file and router from Vue’s default router. It’s completely okay to not understand all of this at once!
After that, you’re creating a Vue instance using the new Vue() and targeting the #app HTML element. You’re also passing in the component App which you imported from the App.vue file. So, basically you’re trying to render App component inside the #app target element. Let’s dig it a bit deeper. Open the App.vue file:
<template>
<div id="app">
<img src="./assets/logo.png">
<router-view />
</div>
</template>
<script>
export default {
name: "App"
};
</script>
Code language: HTML, XML (xml)
In the above code, <template> is used to define a component. It must have one and only one <div> element inside it. You’ll notice there’s an image link and a <router-view/>. <router-view/> is something that represents what components are shown and in which routes. You’ll learn more about components and routes later in details. For now, just remove this line of code to remove the image and save the file.
<img src="./assets/logo.png">
Code language: HTML, XML (xml)
Now, go to router/index.js file and open it:
import Vue from ‘vue’;
import Router from ‘vue-router’;
import HelloWorld from ‘@/components/HelloWorld’;
Vue.use(Router);
export default new Router({
routes: [
{
path: ‘/’,
name: ‘HelloWorld’,
component: HelloWorld
}
] });
Code language: JavaScript (javascript)
In this code, you can see that HelloWorld is being imported and is being passed as a component to “/” route path. Which means, at the home page, the content of the HelloWorld component will be shown. So now, let’s open the src/components/Helloworld.vue . In this file, delete everything and replace with the following code:
<template>
<div>{{ message }}</div>
</template>
<script>
export default {
name: "HelloWorld",
data() {
return {
message: "Hello World!"
};
}
};
</script>
Code language: HTML, XML (xml)
In the above code, data() is a function inside which you can keep multiple variables with some default values. Of course, you can modify the values as per your or user’s necessity. In this case, data function has only one variable called message with a string value assigned as “Hello World”.
Note: This is not the only way you can define variables or get values to be used in your template. You can also get values through computed methods, which you'll see later. In computed methods, you get values though getters. You can get the values of variables using getters, which store the values in the store using vuex.