Passing some props from one component to another hasn’t really changed in the Composition API. However, the way how we receive the props has changed.
Consider the following example where we pass some prop from a component named ParentComponent to a component named ChildComponent. The ChildComponent will receive some text data to be shown inside a paragraph.
//ParentComponent.vue
<script setup>
import ChildComponent from './ChildComponent.vue';
</script>
<template>
<div>
<h2>Parent component</h2>
<ChildComponent content="Some content for the child component"/>
</div>
</template>
Code language: HTML, XML (xml)
//ChildComponent.vue
<script setup>
const props = defineProps({
content: {
type: String,
default: 'No content found!'
}
})
</script>
<template>
<div>
<p>
{{ content }}
</p>
</div>
</template>
Code language: HTML, XML (xml)
As you can see, we no more use the prop: [] property to receive props. Instead, we have a global method available named defineProps(). We can use this method to either receive some props directly without any configuration:
const props = defineProps(['content'])
Code language: JavaScript (javascript)
Or we can receive props with some additional configuration like default values, their types, etc:
const props = defineProps({
content: {
type: String,
default: 'Some default value here!'
}
})
Code language: JavaScript (javascript)
While using the props in the template, we can either use the prop name directly:
<p>
{{ content }}
</p>
Code language: HTML, XML (xml)
Or we can access through the props property:
<p>
{{ props.content }}
</p>
Code language: HTML, XML (xml)
The output of the above code would look like this:
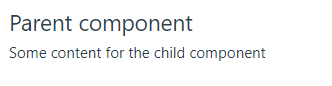
If you have any suggestions or feedback, let me know in the comments section below!